If a developer claimed they’ve never had a brush with burnout, it’s either they’re superhuman or they lied. But let’s be real. It’s most likely the latter.
The dynamic world of coding chaos is never easy, especially with those impossible deadlines, poor project timeline estimates, chaotic app development plans, and miss-release schedules. A continuous case like this pushes developers to work beyond what is physically and mentally sustainable.
That’s where the developer burnout comes in.
Endless exhaustion, no productivity, constant negativism– you might think it’s just one of those days that you got super lazy. But turns out it lasts longer than expected.
Recent Gallup surveys reveal most workers (about 76%) experience burnout. And if you’re one of them, there are some practices that can help you bring the spark back and deal with it. We’ll also include statistics from the State of Developer Wellness Report 2024 to support our points and provide additional insights into effective strategies for overcoming burnout.
How Do You Know You Have a Developer Burnout?
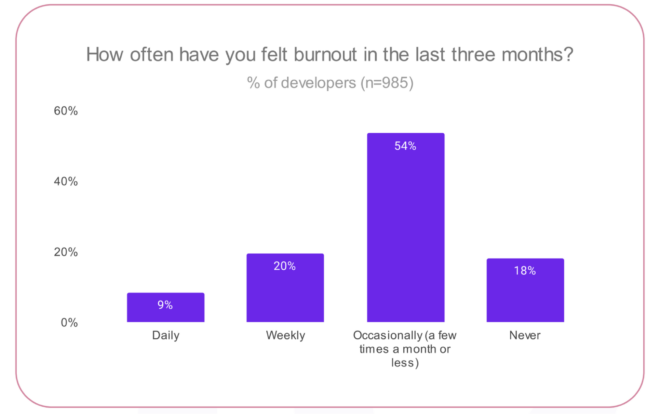
It may start when you notice a decline in your enthusiasm for coding, a constant feeling of exhaustion even after a long, good night’s sleep, or a growing sense of cynicism towards your work and projects.
In fact, over 23% of developers feel like they don’t even have a sense of purpose. Each new task feels like a huge chore, and they find it increasingly difficult to summon the motivation to tackle it.
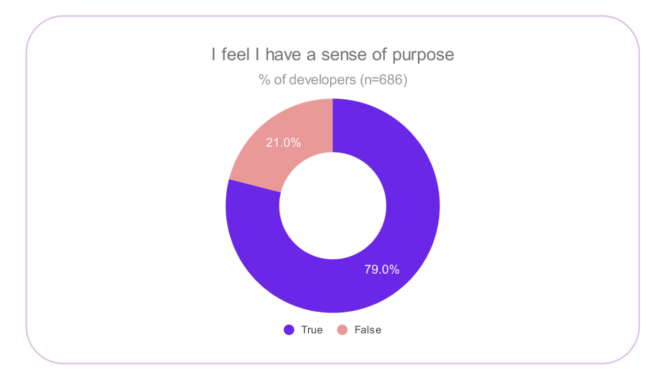
Burnout tends to persist over time. It affects your performance consistently across various tasks and projects. It can manifest physically, such as headaches, muscle tension, or even illness due to chronic stress.
However, you might still enjoy coding. But over time, you find yourself always struggling to muster the energy to engage in it. The work feels meaningless, and every day starts to feel the same.
Meanwhile, feeling lazy might be more sporadic and not necessarily tied to specific circumstances. When you feel lazy, you might experience a temporary lack of motivation or energy, often seeking immediate gratification or avoiding tasks altogether, but without the underlying sense of constant emotional exhaustion.
Burnout typically leads to a decline in the quality of your work, as well as increased errors and difficulty concentrating. If you notice a significant drop in your performance despite your best efforts, it could be a sign of burnout rather than laziness.
How to Deal With Developer Burnout?
The best way is always to seek professional help. But, if you’re looking for immediate strategies to cope with developer burnout, these tips might be helpful to make it less painful and make everything more manageable.
Review and find the trigger
Take some time to reflect on your recent experiences at work and how they have impacted your well-being. Consider moments when you felt particularly stressed, overwhelmed, or demotivated.
The report shows that as many as 76.5% of surveyed developers make time to check on their health and well-being regularly. You can start by looking for patterns or recurring themes in your experiences.
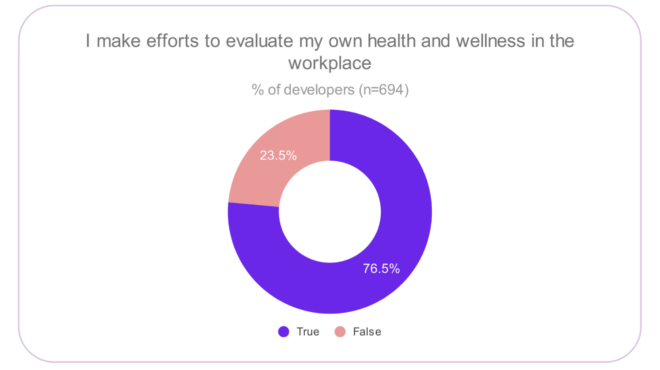
Are there specific tasks, projects, or situations that consistently trigger feelings of burnout? Am I feeling overwhelmed or drained? Am I giving myself enough time for self-care?
Don’t forget the internal factors. Are there any personal habits or thought patterns that might be contributing to your burnout? For example, perfectionism, over-commitment, or difficulty setting boundaries.
When you know the trigger, it will be much easier to gain a better understanding of the factors contributing to your stress and exhaustion.
Go to your safe place
Everyone has their own safe place. Some prefer to do their hobbies, while others like to talk it out to their closest friends or families.
Your safe place is a space or activity where you feel calm, relaxed, and free from stress. It’s a place where you can recharge and rejuvenate your mind and body.
Once you’ve identified your safe place or activity, prioritize making time for it regularly, especially when you’re feeling overwhelmed or burnt out. Set boundaries to protect this time and ensure that you have dedicated opportunities to recharge and take care of yourself.
Prioritize work-life balance
Developer burnout often starts when you neglect your personal needs and well-being in favor of work demands. This imbalance can lead to chronic stress, exhaustion, and feelings of disillusionment with work.
Fortunately, more and more tech companies are aware of the importance of this work-life balance and are implementing initiatives and policies to support their employees’ well-being.
The same report says that at least 77% of companies accommodate developers’ timeout requests. It’s easier for developers to take the necessary breaks and recharge.
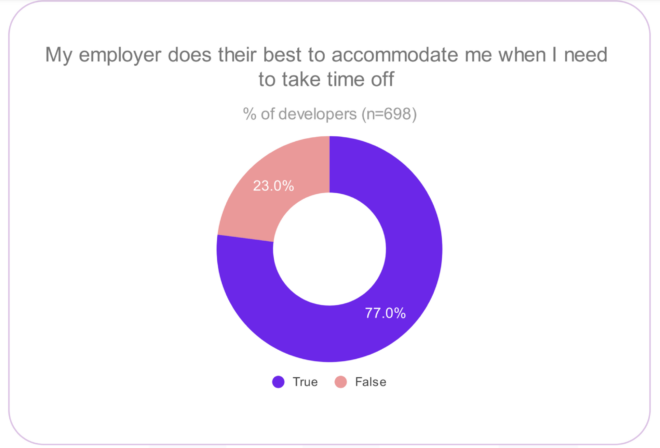
Establish clear boundaries between work and personal life to prevent one from encroaching on the other. Define your specific work hours and commit to unplugging from work-related tasks during personal time.
Understand which tasks and activities are most important to you and allocate your time and energy accordingly. Communicate these boundaries to colleagues and supervisors to ensure they are respected.
Learn to say “no”
Some developers struggle with people-pleasing tendencies, feeling obligated to say “yes” to every request or task that comes their way. This behavior can stem from a desire to be liked, a fear of disappointing others, or a belief that saying “no” is selfish or unprofessional. If you say yes to everything you’ve been asked for, then get ready to feel overworked— just like one-third of other developers.
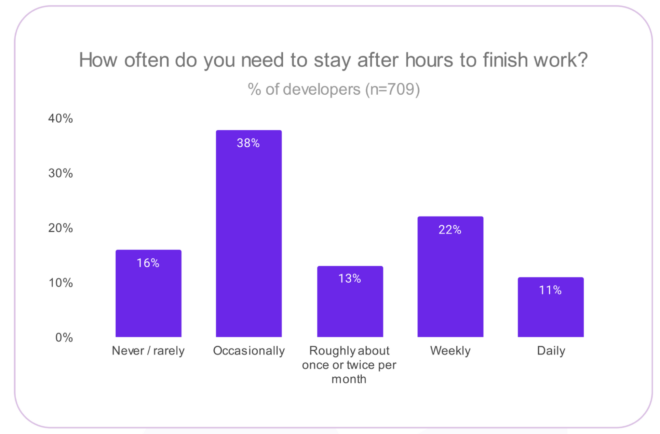
Remember, you’re a developer, not a superhero. You have limits and boundaries that need to be respected, both by yourself and by others. Constantly saying “yes” to everything can lead to burnout and undermine your ability to perform at your best.
Learning to say “no” is not about being selfish or uncooperative. It means filtering which tasks you really have to do and which ones are not even your responsibilities. All of this is for the sake of your well-being and effectiveness.
Work smarter, not harder
Writing good code takes time and a lot of thinking. Contrary to popular stereotypes, effective developers are not the ones who live and breathe code, stay awake all night to resolve bugs, and memorize their code extensively.
An effective developer works smartly. They focus on solving the right problems in the right way. So, working harder doesn’t always equate to working smarter. Use tools that can streamline the whole process and eliminate those mundane, repetitive tasks.
For example, using version control systems like Git allows developers to manage code changes efficiently, collaborate seamlessly with team members, and track project history effectively.
Integrated development environments (IDEs) such as Visual Studio Code or IntelliJ IDEA offer features like code completion, syntax highlighting, and debugging tools that improve productivity and accuracy.
Wrapping Up
Developer burnout is real. All those lines of code mean nothing if the developers behind them are exhausted, demotivated, and struggling to maintain their passion for their work.
Like the small beetles of life, the small symptoms of burnout can continually become stronger and stronger if we don’t interrupt them. By catching the mild feelings, we give ourselves the time for preventive action. If you want to delve deeper into developer burnout and see the statistics firsthand, download the State of Developer Wellness Report 2024.
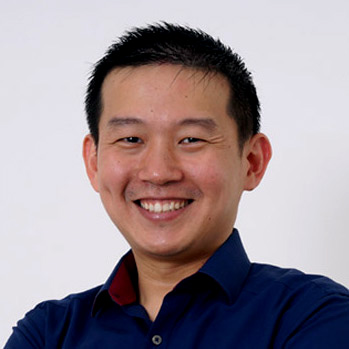